React实现实时搜索查询,快速选择
效果查看
思路分析
原型(mock)
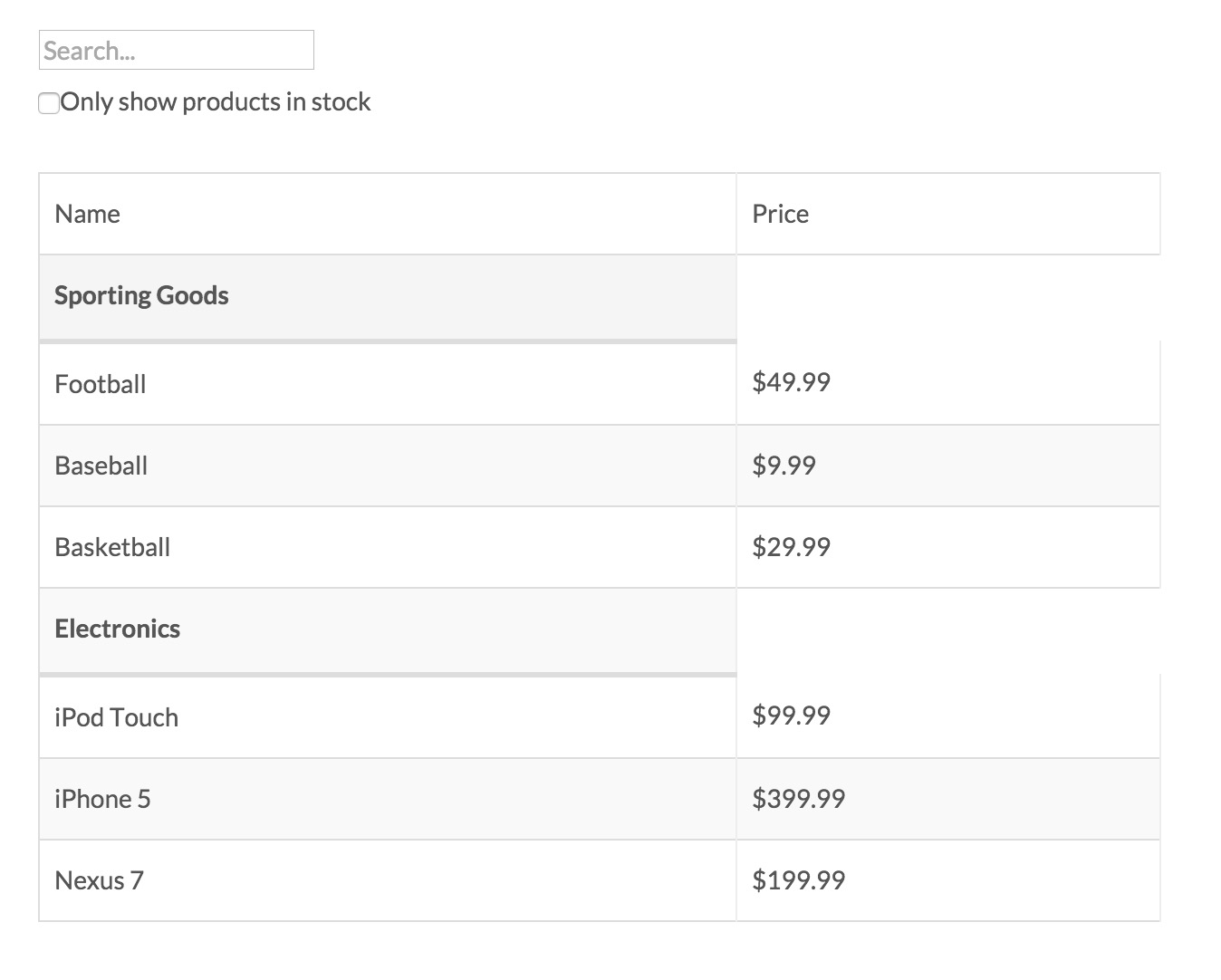
拆分原型为一个组件树
遵循单一功能原则,指的是,理想状态下一个组件应该只做一件事,假如它功能逐渐变大就需要被拆分成更小的子组件。
原型界面和数据模型在 信息构造 方面都要一致 (JSON数据模型与UI组件结构一致性)
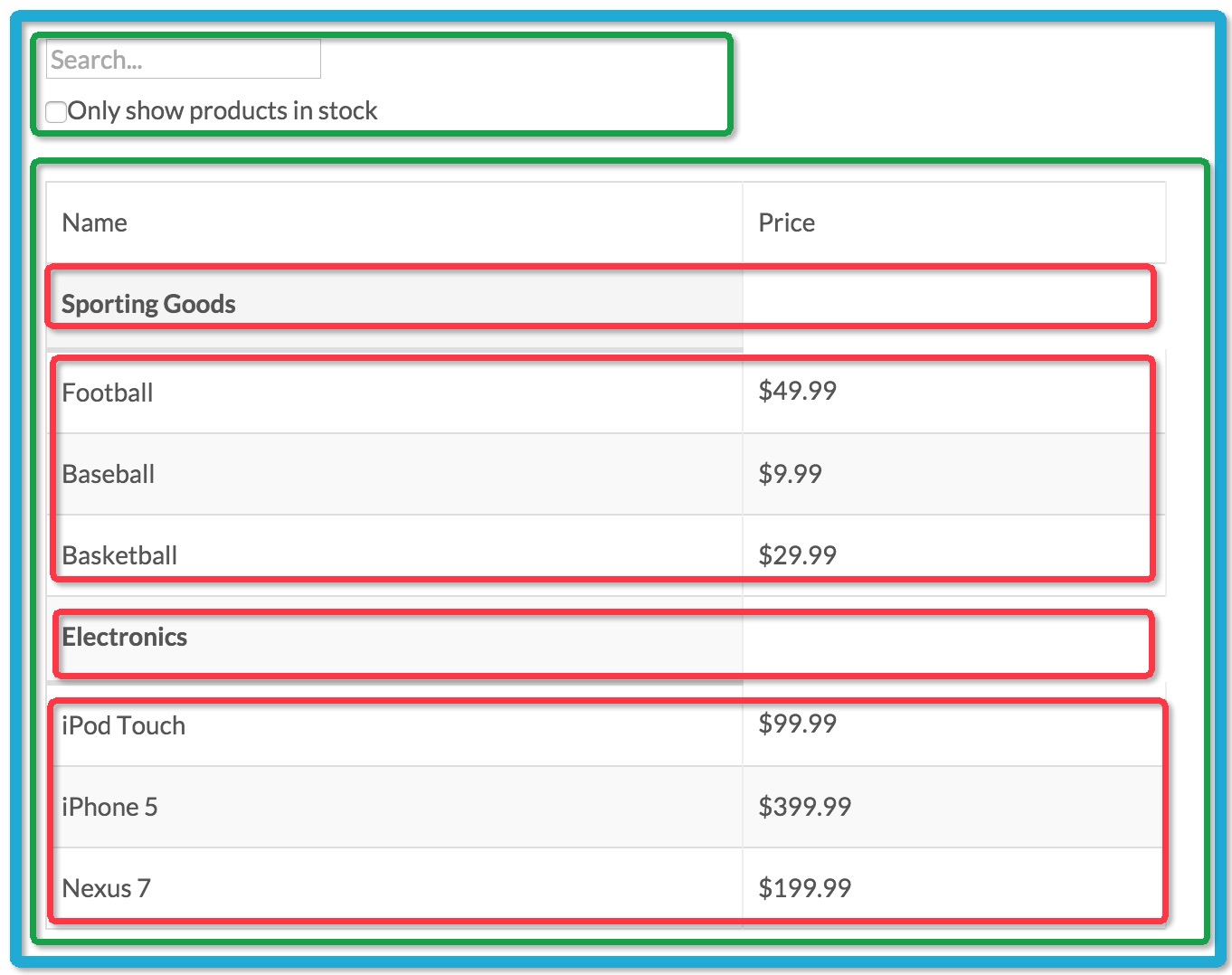
将原型划分为5个组件
- FilterableProductTable 包含整个例子的容器
- SearchBar 接受所有 用户输入( user input )
- ProductTable 根据 用户输入( user input ) 过滤和展示 数据集合( data collection )
- ProductCategoryRow 为每个 分类( category ) 展示一列表头
- ProductRow 为每个 产品( product ) 展示一列
创建静态版本
通过组件树,简单的将数据模型渲染到UI上。
构建一个静态的版本仅需要大量的输入,而不需要思考。
创建一个渲染数据模型的应用的静态版本,你将会构造一些组件,这些组件重用其它组件,并且通过 props 传递数据。
props 是一种从父级向子级传递数据的方式。
识别最小(最完整)代表UI的state
为了使 UI 可交互,需要能够触发底层数据模型的变化。 React 通过 state 使这变得简单。
state 是:
- 用户输入的搜索文本
- 复选框的值
确认state生命周期
React 中数据是沿着组件树从上到下单向流动的。
我们在应用中应用这个策略:
- ProductTable 需要基于 state 过滤产品列表,SearchBar 需要显示搜索文本和复选框状态。
- 共同拥有者组件是 FilterableProductTable 。
- 理论上,过滤文本和复选框值位于 FilterableProductTable 中是合适的。
给 FilterableProductTable 添加 getInitialState() 方法,该方法返回 {filterText: ‘’, inStockOnly: false} 来反映应用的初始化状态。
传递 filterText 和 inStockOnly 给 ProductTable 和 SearchBar 作为 prop 。
使用这些 props 来过滤 ProductTable 中的行,设置在 SearchBar 中表单字段的值。
添加反向数据流
无论何时用户改变了表单,都要更新 state 来反映用户的输入。
由于组件只能更新自己的 state , FilterableProductTable 将会传递一个回调函数给 SearchBar ,此函数将会在 state 应该被改变的时候触发。
使用 input 的 onChange 事件来监听用户输入,从而确定何时触发回调函数。
FilterableProductTable 传递的回调函数将会调用 setState() ,然后应用将会被更新。
源代码
相关依赖
- https://cdnjs.cloudflare.com/ajax/libs/react/0.14.7/react.js
- https://cdnjs.cloudflare.com/ajax/libs/react/0.14.7/react-dom.js
- https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.6.15/browser.js
- https://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.0/jquery.min.js
1 | var PRODUCTS = [ |
相关知识总结
- 组件划分遵循单一功能原则,由上往下,由大到小依次组件化;
- React应用搭建三部曲
- 通过props搭建基于数据结构和UI的静态版本;
- 识别最小、最完整state,确认state生命周期(getInitialState() 初始化state,更新部分由state代替props);
- 添加反向数据流(回调,数据更新setState())。
- props 是一种从父级向子级传递数据的方式。
- 无论何时用户改变了表单,都要更新 state 来反映用户的输入